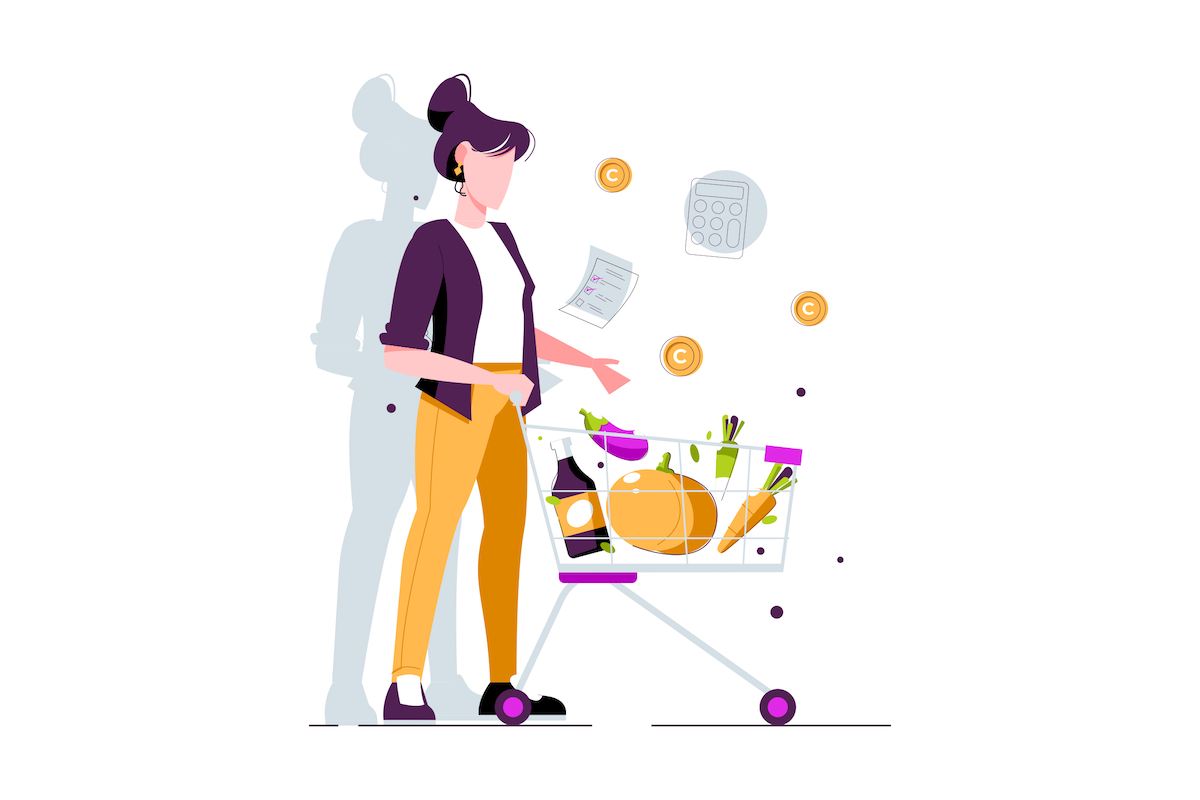
This article will show how the Vue Composition API is a great way to make your code more readable and maintainable. With the composition API introduced in Vue 3, handling of methods and component state is made more accessible.
The Composition API is a new and optional way of creating and organizing components in a Vue 3 application. It allows reactive component logic to be defined more intuitively by allowing all the code for a specific feature (search, for example) to be grouped. Using the Vue Composition API will make your application more scalable and reusable between several components.
In this article, we’ll build a simple shopping List app with the Vue Composition API.
You can check out a live demo of the app we’re building.
Prerequisites
For this tutorial, you’ll need:
Setting Up the Vue Application
Now let’s start by installing Vue Cli:
npm install -g vue-cli
This command will install Vue globally.
We’ll use the Vue CLI to build a simple application. To do that, open up your terminal and type the following:
vue create vueshoppinglist
After installation, move into the folder using the cd vueshoppinglist
and run npm run serve
.
This starts a development server that allows you to view your app on localhost:8080.
It’s now time to set up a nice Vue project.
The Vue Composition API
To Install the Composition API from the root of your project, run the following:
npm install --save @vue/composition-api
After successfully installing, we’ll import it into our project.
Modify src/main.vue
to register the Composition API globally in our application, so that we can use it in all our application components:
import Vue from 'vue'
import App from './App.vue'
import VueCompositionApi from '@vue/composition-api'
Vue.config.productionTip = false
Vue.use(VueCompositionApi)
new Vue({
render: h => h(App),
}).$mount('#app')
Building Out the User Interface
We’ll need a component that will house the UI of our app. Create a new ShoppingList.vue
component in the src/components/
directory and paste the following into the file:
<template>
<section>
<div class="form-container">
<h2>Add Item</h2>
<form>
<div>
<label>Product name</label>
<br />
<input type="text" />
</div>
<div>
<button type="submit" class="submit">Add Item</button>
</div>
</form>
</div>
<div class="list-container">
<ul>
<li>
Shopping List app
<span style="float:right;padding-right:10px;">
<button>X</button>
</span>
</li>
</ul>
</div>
</section>
</template>
<script>
export default {};
</script>
<style scoped>
input {
width: 20%;
height: 30px;
border: 2px solid green;
}
.submit {
margin: 10px;
padding: 10px;
border-radius: 0px;
border: 0px;
background: green;
color: white;
}
ul li {
list-style: none;
border: 2px solid green;
width: 30%;
margin-top: 10px;
}
</style>
The code snippet above is the initial boilerplate of our UI. We’ll now import our new component ShoppingList.vue
to App.vue
as shown below:
<template>
<div id="app">
<img alt="Shoppingd List" src="./assets/shopping.png">
<shopping-list msg="Welcome to Your Vue.js App"/>
</div>
</template>
<script>
import ShoppingList from './components/ShoppingList.vue'
export default {
name: 'App',
components: {
ShoppingList
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
Continue reading Building a Shopping List App with the Vue Composition API on SitePoint.
No comments:
Post a Comment