Have you tried to find functionality for a specific purpose in WordPress but failed to find a plugin or widget that does your work? This post walks through how you can create a WordPress widget, even if you have limited knowledge of coding.
WordPress Widgets are blocks of code — static, dynamic, or a combination of both — which can be added to any specific area of your WordPress site. There are many built-in widgets in WordPress — such as tag cloud and categories — but this post helps you create a widget from scratch in a short time. It will probably take you longer to finish reading this article than to create the widget after you gain the knowledge!
For illustration purposes, we’re looking at creating a widget with dynamic content in the form of real time data of cricket matches. The purpose of creating dynamic content is to familiarize you with various functions that are associated with WordPress Widgets.
Pre Requisites
Before you start any code, please make sure you back up your WordPress code, as you may end up with a white screen of death. Alternately, you may want to explore version control for WordPress with Git.
Further, it’s recommended that you have some knowledge of OOP in PHP before attempting to create a widget.
This tutorial has been created on WordPress version 4.9.8.
Where to Put the Code?
Before we discuss the nuances of developing a WordPress Widget, let’s consider where to keep the code. The answer to that lies in the purpose for developing the widget. In case you want the widget to appear in a specific theme only, you can put the code in the theme’s functions.php
file. However, this makes the widget accessible only if the theme is active.
This tutorial focuses on creating a widget which can be accessed throughout themes on your website. Hence, we would create a new plugin, which houses the widget that shows a list of live cricket matches from an API.
Widget Development Basics
To create a widget in WordPress, you need to extend the WP_Widget
class. Within your widget class, you can create a list of functions:
- the constructor function
- the
widget
function to display the widget contents - the
form
function if you need to define a form that takes input - the
update
function if you need to update the settings of the widget.
In the example of displaying cricket feeds, we need to define only the first two functions. Further, once we’ve created the widget class, we need to register it with the register_widget
function.
Let’s now move on to creating a basic plugin in WordPress and then use an API to generate dynamic content.
The Basics: Hello World Widget
Before you create the widget, an empty plugin needs to be created. Head on to the /wp-content/plugins/
and create a new directory with the slug of the name of your plugin. Within this directory, create an index.php
file with the following content.
Define an Empty Plugin
<?php
/*
Plugin Name: Live Score Custom
Plugin URI: https://www.sitepoint.com/
Description: Get and display sports feeds
Version: 1.0
Author: Shaumik
Author URI: https://www.sitepoint.com/
License: GPL2
*/
?>
This is essentially a blank PHP file with comments, as suggested on the WordPress Codex page on creating a new plugin. These comments are read by WordPress when displaying information about the plugin on WP Admin.
Once you’ve created the empty plugin with comments, you can see that your plugin is active on the list of plugins on WP Admin. Activate the plugin before proceeding further.
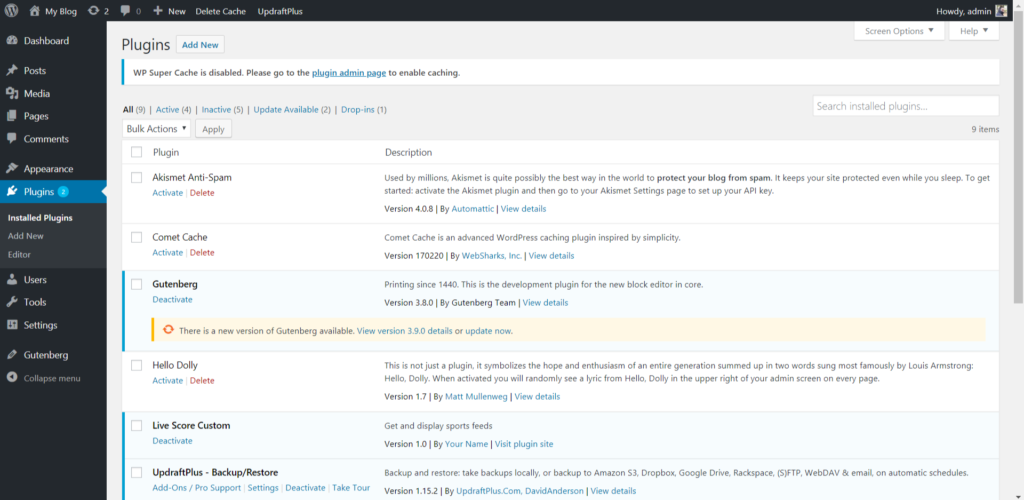
Define a Widget within the Plugin
The next step is to create a sample widget code. As discussed earlier, we would be extending the WP_Widget
class. After defining the class, we’ll register the widget using the register_widget
method:
class My_Custom_Widget extends WP_Widget {
public function __construct() {
}
public function widget( $args, $instance ) {
// Define the widget
}
}
// Register the widget
function my_register_custom_widget() {
register_widget( 'My_Custom_Widget' );
}
add_action( 'widgets_init', 'my_register_custom_widget' );
Now that our skeleton code is ready, let’s fill in the constructor and widget
functions:
public function __construct() {
// Define the constructor
$options = array(
'classname' => 'custom_livescore_widget',
'description' => 'A live score widget',
);
parent::__construct(
'live_score_widget', 'Live Score Widget', $options
);
}
The constructor can be extended from the parent constructor. In the options, we can also provide a CSS class
that’s added to the entire widget element in the HTML DOM when being displayed. Next, let’s define the widget display options:
public function widget( $args, $instance ) {
// Keep this line
echo $args['before_widget'];
echo $args['before_title'] . apply_filters( 'widget_title', 'Live Criket Matches' ) . $args['after_title'];
echo 'Hello, World!';
// Keep this line
echo $args['after_widget'];
}
In the widget
class, we define what needs to be displayed in the widget. First, we add a widget title, “Live Cricket Matches”, and then a simple “Hello, World!”
Once the code has been saved, let’s head back to Appearance > Widgets in WP Admin and add the newly created widget to a part of the page.
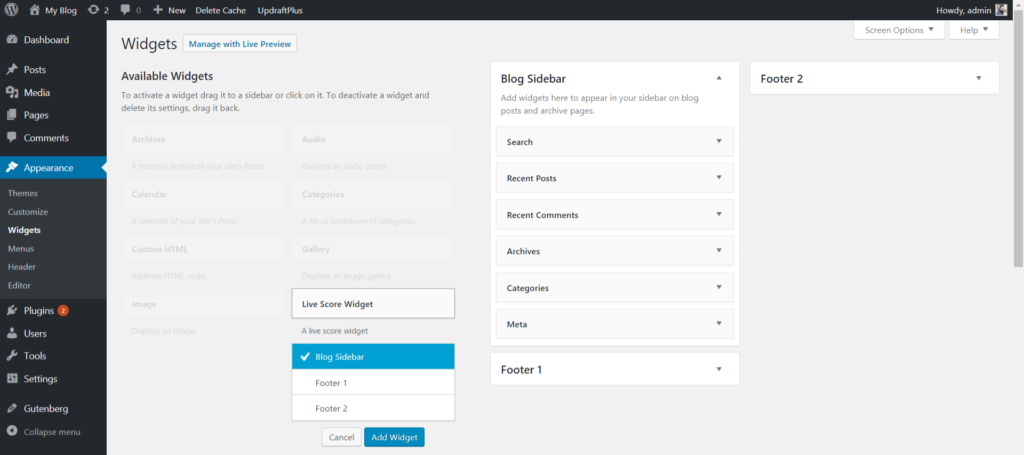
Once you’ve added the widget, you can refresh your home page and check if the widget has appeared.
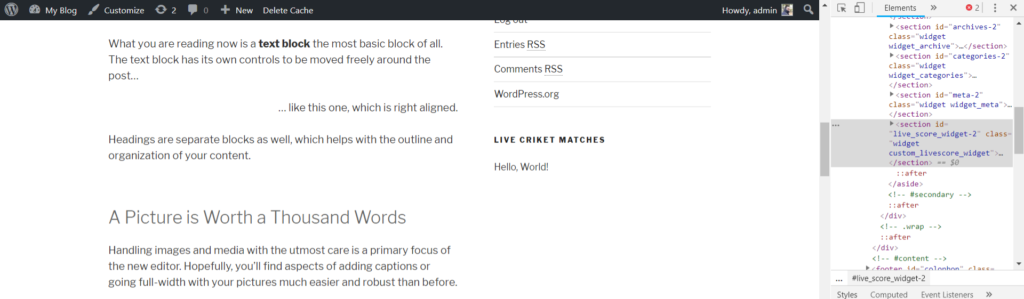
You may notice that the class in which we defined the widget has been added to the widget element. You can change the style of the widget if required. If you’re interested, check out this tutorial on how to customize the style of a WordPress theme.
The post Create a Dynamic Widget in WordPress in Ten Minutes appeared first on SitePoint.
No comments:
Post a Comment